Arduino Uno + CMPS14
It is pretty essential to have a tilt compensated compass module on a boat that heels. The CMPS14 compass is an example which met this requirement. Compared to the CMPS12 the compass has upgraded from the Bosch BNO055 to the BNO080. The automatic calibration was removed which I feel an improvement for early compass readings. The accelerometer now gives Linear Accelerator vector without gravity, which is what I want.
The last script is some calibration code.
Video
Please click thumbnail image to start the video
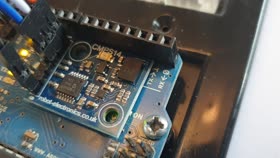
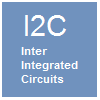
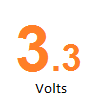
Photo
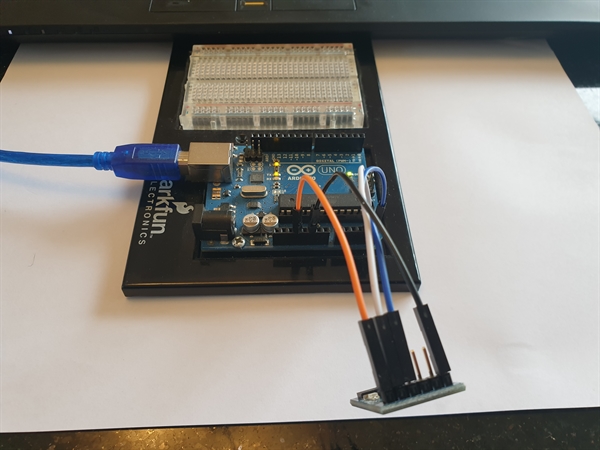
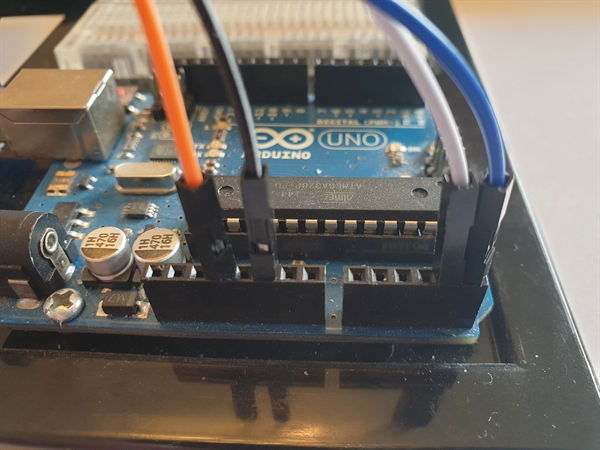
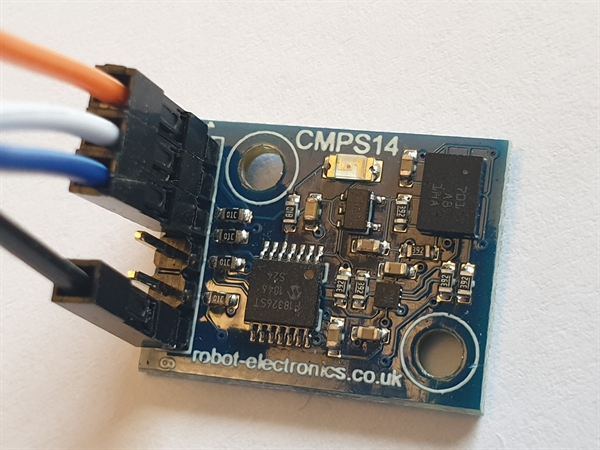
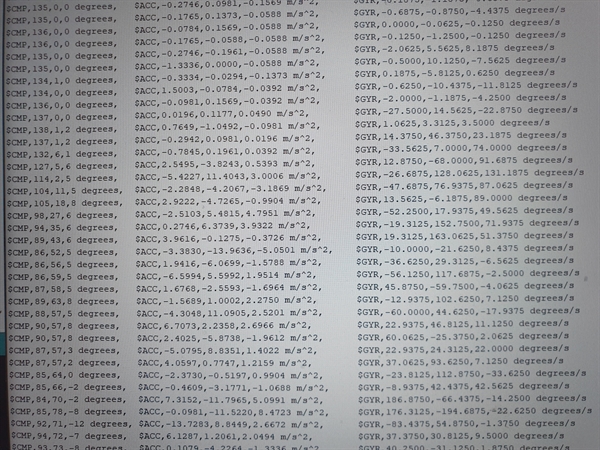
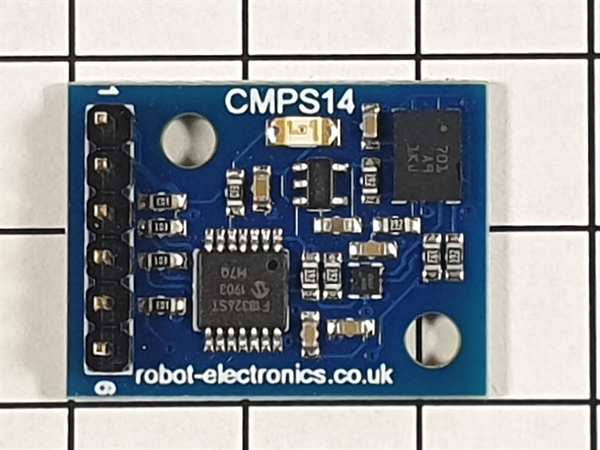
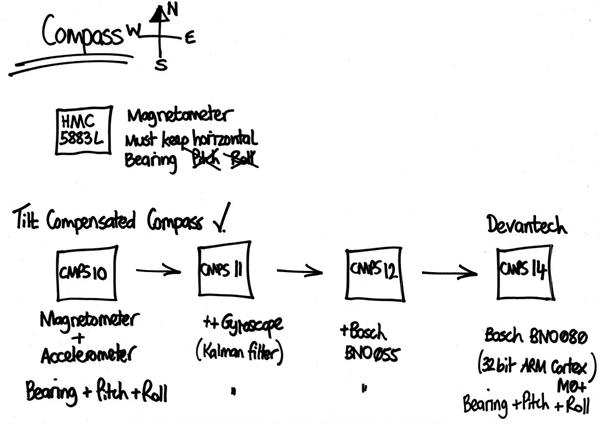
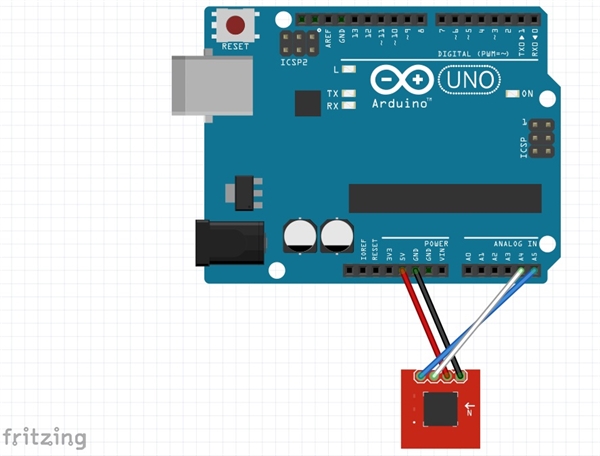
Sketch
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Digital Compass CMPS14 | |
// Copyright (C) 2021 https://www.roboticboat.uk | |
// ccc3d672-cfb3-4df4-b0af-7b80a580dded | |
// | |
// This program is free software: you can redistribute it and/or modify | |
// it under the terms of the GNU General Public License as published by | |
// the Free Software Foundation, either version 3 of the License, or | |
// (at your option) any later version. | |
// | |
// This program is distributed in the hope that it will be useful, | |
// but WITHOUT ANY WARRANTY; without even the implied warranty of | |
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |
// GNU General Public License for more details. | |
// | |
// You should have received a copy of the GNU General Public License | |
// along with this program. If not, see <https://www.gnu.org/licenses/>. | |
// These Terms shall be governed and construed in accordance with the laws of | |
// England and Wales, without regard to its conflict of law provisions. | |
#include <Wire.h> | |
// Register Function | |
// 0 Command register | |
// 1 Compass Bearing as a byte, i.e. 0-255 for a full circle | |
// 2,3 Compass Bearing as a word, i.e. 0-3599 for a full circle, representing 0-359.9 degrees. Register 2 being the high byte | |
// 4 Pitch angle - signed byte giving angle in degrees from the horizontal plane, Kalman filtered with Gyro | |
// 5 Roll angle - signed byte giving angle in degrees from the horizontal plane, Kalman filtered with Gyro | |
// 6,7 Magnetometer X axis raw output, 16 bit signed integer with register 6 being the upper 8 bits | |
// 8,9 Magnetometer Y axis raw output, 16 bit signed integer with register 8 being the upper 8 bits | |
// 10,11 Magnetometer Z axis raw output, 16 bit signed integer with register 10 being the upper 8 bits | |
// 12,13 Accelerometer X axis raw output, 16 bit signed integer with register 12 being the upper 8 bits | |
// 14,15 Accelerometer Y axis raw output, 16 bit signed integer with register 14 being the upper 8 bits | |
// 16,17 Accelerometer Z axis raw output, 16 bit signed integer with register 16 being the upper 8 bits | |
// 18,19 Gyro X axis raw output, 16 bit signed integer with register 18 being the upper 8 bits | |
// 20,21 Gyro Y axis raw output, 16 bit signed integer with register 20 being the upper 8 bits | |
// 22,23 Gyro Z axis raw output, 16 bit signed integer with register 22 being the upper 8 bits | |
//--------------------------------- | |
//Address of the CMPS14 compass on i2c | |
#define _i2cAddress 0x60 | |
#define CONTROL_Register 0 | |
#define BEARING_Register 2 | |
#define PITCH_Register 4 | |
#define ROLL_Register 5 | |
#define MAGNETX_Register 6 | |
#define MAGNETY_Register 8 | |
#define MAGNETZ_Register 10 | |
#define ACCELEROX_Register 12 | |
#define ACCELEROY_Register 14 | |
#define ACCELEROZ_Register 16 | |
#define GYROX_Register 18 | |
#define GYROY_Register 20 | |
#define GYROZ_Register 22 | |
#define ONE_BYTE 1 | |
#define TWO_BYTES 2 | |
#define FOUR_BYTES 4 | |
#define SIX_BYTES 6 | |
//--------------------------------- | |
byte _byteHigh; | |
byte _byteLow; | |
// Please note without clear documentation in the technical documenation | |
// it is notoriously difficult to get the correct measurement units. | |
// I've tried my best, and may revise these numbers. | |
int bearing; | |
int nReceived; | |
signed char pitch; | |
signed char roll; | |
float magnetX = 0; | |
float magnetY = 0; | |
float magnetZ = 0; | |
float accelX = 0; | |
float accelY = 0; | |
float accelZ = 0; | |
// The acceleration along the X-axis, presented in mg | |
// See BNO080_Datasheet_v1.3 page 21 | |
float accelScale = 9.80592991914f/1000.f; // 1 m/s^2 | |
float gyroX = 0; | |
float gyroY = 0; | |
float gyroZ = 0; | |
// 16bit signed integer 32,768 | |
// Max 2000 degrees per second - page 6 | |
float gyroScale = 1.0f/16.f; // 1 Dps | |
void setup() { | |
// Initialize the serial port to the User | |
// Set this up early in the code, so the User sees all messages | |
Serial.begin(9600); | |
// Initialize i2c | |
Wire.begin(); | |
} | |
void loop() { | |
// Read the Compass | |
ReadCompass(); | |
// Read the Accelerator | |
ReadAccelerator(); | |
// Read the Gyroscope | |
ReadGyro(); | |
// Print data to Serial Monitor window | |
Serial.print("$CMP,"); | |
Serial.print(bearing); | |
Serial.print(","); | |
Serial.print(pitch); | |
Serial.print(","); | |
Serial.print(roll); | |
Serial.print(" degrees,"); | |
Serial.print("\t$ACC,"); | |
Serial.print(accelX,4); | |
Serial.print(","); | |
Serial.print(accelY,4); | |
Serial.print(","); | |
Serial.print(accelZ,4); | |
Serial.print(" m/s^2,"); | |
Serial.print("\t$GYR,"); | |
Serial.print(gyroX,4); | |
Serial.print(","); | |
Serial.print(gyroY,4); | |
Serial.print(","); | |
Serial.print(gyroZ,4); | |
Serial.println(" degrees/s"); | |
} | |
int16_t getBearing() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(BEARING_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate full bearing | |
bearing = ((_byteHigh<<8) + _byteLow) / 10; | |
return bearing; | |
} | |
byte getPitch() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(PITCH_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 1 byte from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , ONE_BYTE); | |
// Something has gone wrong | |
if (nReceived != ONE_BYTE) return 0; | |
// Read the values | |
pitch = Wire.read(); | |
return pitch; | |
} | |
byte getRoll() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(ROLL_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 1 byte from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , ONE_BYTE); | |
// Something has gone wrong | |
if (nReceived != ONE_BYTE) return 0; | |
// Read the values | |
roll = Wire.read(); | |
return roll ; | |
} | |
int16_t getgyroX() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(GYROX_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate GryoX | |
return ((_byteHigh<<8) + _byteLow); | |
} | |
int16_t getgyroY() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(GYROY_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate GryoY | |
return ((_byteHigh<<8) + _byteLow); | |
} | |
int16_t getgyroZ() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(GYROZ_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate GryoZ | |
return ((_byteHigh<<8) + _byteLow); | |
} | |
int16_t getAcceleroX() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(ACCELEROX_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate Accelerometer | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
int16_t getAcceleroY() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(ACCELEROY_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate Accelerometer | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
int16_t getAcceleroZ() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(ACCELEROZ_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate Accelerometer | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
int16_t getMagnetX() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(MAGNETX_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate value | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
int16_t getMagnetY() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(MAGNETY_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate value | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
int16_t getMagnetZ() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(MAGNETZ_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return 0;} | |
// Request 2 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , TWO_BYTES); | |
// Something has gone wrong | |
if (nReceived != TWO_BYTES) return 0; | |
// Read the values | |
_byteHigh = Wire.read(); | |
_byteLow = Wire.read(); | |
// Calculate value | |
return (((int16_t)_byteHigh <<8) + (int16_t)_byteLow); | |
} | |
void ReadCompass() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(BEARING_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){bearing = 0; pitch = 0; roll = 0; return;} | |
// Request 4 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , FOUR_BYTES); | |
// Something has gone wrong | |
if (nReceived != FOUR_BYTES) {bearing = 0; pitch = 0; roll = 0; return;} | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
bearing = ((_byteHigh<<8) + _byteLow) / 10; | |
// Read the values | |
pitch = Wire.read(); | |
// Read the values | |
roll = Wire.read(); | |
} | |
void ReadAccelerator() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(ACCELEROX_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){accelX = 0; accelY = 0; accelZ = 0; return;} | |
// Request 6 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , SIX_BYTES); | |
// Something has gone wrong | |
if (nReceived != SIX_BYTES) {accelX = 0; accelY = 0; accelZ = 0; return;} | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
accelX = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * accelScale; | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
accelY = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * accelScale; | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
accelZ = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * accelScale; | |
} | |
void ReadGyro() | |
{ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(GYROX_Register); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){gyroX = 0; gyroY = 0; gyroZ = 0; return;} | |
// Request 6 bytes from CMPS14 | |
nReceived = Wire.requestFrom(_i2cAddress , SIX_BYTES); | |
// Timed out so return | |
if (nReceived != SIX_BYTES) {accelX = 0; accelY = 0; accelZ = 0; return;} | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
gyroX = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * gyroScale; | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
gyroY = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * gyroScale; | |
// Read the values | |
_byteHigh = Wire.read(); _byteLow = Wire.read(); | |
gyroZ = (((int16_t)_byteHigh <<8) + (int16_t)_byteLow) * gyroScale; | |
} | |
void changeAddress(byte i2cAddress, byte newi2cAddress) | |
{ | |
// Reset the address on the i2c network | |
// Ensure that you have only this module connected on the i2c network | |
// The 7 bit i2c address must end with a 0. (even numbers please) | |
// For example changeAddress(0x60, 0x64) | |
// Address 0x60, 1 long flash, 0 short flashes | |
// Address 0x62, 1 long flash, 1 short flashes | |
// Address 0x64, 1 long flash, 2 short flashes | |
// Address 0x66, 1 long flash, 3 short flashes | |
// Address 0x68, 1 long flash, 4 short flashes | |
// Address 0x6A, 1 long flash, 5 short flashes | |
// Address 0x6C, 1 long flash, 6 short flashes | |
// Address 0x6E, 1 long flash, 7 short flashes | |
// Begin communication | |
Wire.beginTransmission(i2cAddress); | |
Wire.write(CONTROL_Register); | |
Wire.write(byte(0xA0)); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
//Wait 100ms | |
delay(100); | |
// Begin communication | |
Wire.beginTransmission(i2cAddress); | |
Wire.write(CONTROL_Register); | |
Wire.write(byte(0xAA)); | |
// End the transmission | |
nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return;} | |
//Wait 100ms | |
delay(100); | |
// Begin communication | |
Wire.beginTransmission(i2cAddress); | |
Wire.write(CONTROL_Register); | |
Wire.write(byte(0xA5)); | |
// End the transmission | |
nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return;} | |
//Wait 100ms | |
delay(100); | |
// Begin communication | |
Wire.beginTransmission(i2cAddress); | |
Wire.write(CONTROL_Register); | |
Wire.write(newi2cAddress); | |
// End the transmission | |
nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){return;} | |
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// Arduino UNO with CMPS14 i2c (calibration) | |
// Copyright (C) 2022 https://www.roboticboat.uk | |
// 6ab8ac9b-75f3-45dd-ace5-fda74dc22fb1 | |
// | |
// This program is free software: you can redistribute it and/or modify | |
// it under the terms of the GNU General Public License as published by | |
// the Free Software Foundation, either version 3 of the License, or | |
// (at your option) any later version. | |
// | |
// This program is distributed in the hope that it will be useful, | |
// but WITHOUT ANY WARRANTY; without even the implied warranty of | |
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |
// GNU General Public License for more details. | |
// | |
// You should have received a copy of the GNU General Public License | |
// along with this program. If not, see <https://www.gnu.org/licenses/>. | |
// These Terms shall be governed and construed in accordance with the laws of | |
// England and Wales, without regard to its conflict of law provisions. | |
// Arduino 1.8.19 IDE | |
#include <Wire.h> | |
#define _i2cAddress 0x60 | |
#define calibrationQuality 0x1E | |
// https://stackoverflow.com/questions/111928 (nice trick) | |
#define BYTE_TO_BINARY_PATTERN "%c%c%c%c%c%c%c%c" | |
#define BYTE_TO_BINARY(byte) \ | |
(byte & 0x80 ? '1' : '0'), \ | |
(byte & 0x40 ? '1' : '0'), \ | |
(byte & 0x20 ? '1' : '0'), \ | |
(byte & 0x10 ? '1' : '0'), \ | |
(byte & 0x08 ? '1' : '0'), \ | |
(byte & 0x04 ? '1' : '0'), \ | |
(byte & 0x02 ? '1' : '0'), \ | |
(byte & 0x01 ? '1' : '0') | |
// Timer | |
unsigned long mytime; | |
// Character array | |
char Message[100]; | |
void setup() { | |
// Keep the User informed | |
Serial.begin(9600); | |
// Set i2c network | |
Wire.begin(); | |
Serial.println("----------------------"); | |
Serial.println(" Calibrate CMPS14"); | |
Serial.println("----------------------"); | |
Serial.print("Software version v"); | |
Serial.println(getVersion()); | |
CalibrationQuality(); | |
Serial.println("Just rotate the CMPS14 around for 20 seconds"); | |
Countdown(); | |
CalibrationQuality(); | |
Serial.println(""); | |
Serial.println("Waiting for User input in textbox above next to send button"); | |
Serial.println("Enter 'c' to see calibration"); | |
Serial.println("Enter 'g' to calibrate the gyroscope"); | |
Serial.println("Enter 'a' to calibrate accelometer"); | |
Serial.println("Enter 'm' to calibrate magnetometer"); | |
Serial.println("Enter 's' to save calibration - manual now"); | |
Serial.println("Enter 'p' to start periodic saves"); | |
Serial.println("Enter 'x' to stop periodic saves"); | |
} | |
void loop() { | |
// Timer | |
mytime = millis(); | |
// Check the Serial port | |
while(Serial.available()) | |
{ | |
// Read User input | |
byte a = Serial.read(); | |
// Do we start the calibration? | |
if (a == 'm' || a == 'a' || a == 'g' || a == 'p' || a == 'x'){ | |
// Configuation byte | |
writeToCMPS14(byte(0x98)); | |
writeToCMPS14(byte(0x95)); | |
writeToCMPS14(byte(0x99)); | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Want the Command Register | |
Wire.write(byte(0x00)); | |
// Send some data | |
switch (a){ | |
case 'm': | |
Serial.println("Magnet..."); | |
Wire.write(byte(B10000001)); | |
Serial.println("Rotate the CMPS14 randomly around for 20 seconds"); | |
Countdown(); | |
CalibrationQuality(); | |
break; | |
case 'a': | |
Serial.println("Accel..."); | |
Wire.write(byte(B10000010)); | |
Serial.println("Rotate in differnt 90 degrees and keep steady for a while"); | |
Countdown(); | |
CalibrationQuality(); | |
break; | |
case 'g': | |
Serial.println("Gyro... Keep the CMPS14 stationary"); | |
Wire.write(byte(B10000100)); | |
Countdown(); | |
CalibrationQuality(); | |
break; | |
case 'p': | |
Serial.println("Periodic automatic save of calibration data"); | |
Wire.write(byte(B10010000)); | |
CalibrationQuality(); | |
break; | |
case 'x': | |
Serial.println("Stop auto calibration"); | |
Wire.write(byte(B10000000)); | |
CalibrationQuality(); | |
break; | |
} | |
Serial.println(""); | |
Serial.println("Waiting for User input in textbox above next to send button"); | |
Serial.println("Enter 'c' to see calibration"); | |
Serial.println("Enter 'g' to calibrate the gyroscope"); | |
Serial.println("Enter 'a' to calibrate accelometer"); | |
Serial.println("Enter 'm' to calibrate magnetometer"); | |
Serial.println("Enter 's' to save calibration - manual now"); | |
Serial.println("Enter 'p' to start periodic saves"); | |
Serial.println("Enter 'x' to stop periodic saves"); | |
} | |
// Show calibration quality | |
if (a == 'c'){ | |
CalibrationQuality(); | |
} | |
// Store the calibration | |
if (a == 's'){ | |
writeToCMPS14(byte(0xF0)); | |
writeToCMPS14(byte(0xF5)); | |
writeToCMPS14(byte(0xF6)); | |
// Update the User | |
Serial.println("Saved"); | |
} | |
// Reset the calibration | |
if (a == 'r'){ | |
writeToCMPS14(byte(0xE0)); | |
writeToCMPS14(byte(0xE5)); | |
writeToCMPS14(byte(0xE2)); | |
// Update the User | |
Serial.println("Reset"); | |
delay(500); | |
} | |
} | |
// Wait 100ms so the output is slower | |
delay(100); | |
} | |
void writeToCMPS14(byte n){ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Want the Command Register | |
Wire.write(byte(0x00)); | |
// Send some data | |
Wire.write(n); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){ | |
Serial.println("communication error"); | |
} | |
else | |
{ | |
Serial.println("OK"); | |
} | |
// Wait 100ms | |
delay(100); | |
} | |
void CalibrationQuality(){ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Tell register you want some data | |
Wire.write(calibrationQuality); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0) return; | |
// Request 1 byte from CMPS14 | |
int nReceived = Wire.requestFrom(_i2cAddress, 1); | |
// Timed out so return | |
if (nReceived != 1) return; | |
// Read the values | |
byte calibration = Wire.read(); | |
sprintf(Message,"Calibration " BYTE_TO_BINARY_PATTERN "\n", BYTE_TO_BINARY(calibration)); | |
Serial.print(Message); | |
} | |
byte getVersion(){ | |
// Begin communication with CMPS14 | |
Wire.beginTransmission(_i2cAddress); | |
// Want the Command Register | |
Wire.write(byte(0x00)); | |
// Send some data | |
Wire.write(byte(0x11)); | |
// End the transmission | |
int nackCatcher = Wire.endTransmission(); | |
// Return if we have a connection problem | |
if(nackCatcher != 0){ | |
Serial.println("Communication error"); | |
} | |
// Request 1 byte from CMPS14 | |
int nReceived = Wire.requestFrom(_i2cAddress, 1); | |
// Read the values | |
byte ver = Wire.read(); | |
return ver; | |
} | |
void Countdown(){ | |
int i; | |
for (i=20; i>0; i--){ | |
Serial.print(i,DEC); | |
Serial.print(" "); | |
delay(1000); | |
} | |
Serial.println(""); | |
} |