SD card read writer
Example code of the Raspberry Pi Pico W connected to a Secure Digital (SD) card reader.
Connections
Description | Colour | Pico W | SD card |
---|---|---|---|
Power 3v | Orange | pin36 3V3(OUT) | 3.3v |
Ground | Black | pin3 GND | GND |
SPI | Blue | pin14 GP10 SPI1 SCK | SCK |
SPI | Yellow | pin15 GP11 SPI1 TX | MOSI |
SPI | Green | pin16 GP12 SPI1 RX | MISO |
SPI | White | pin17 GP13 SPI1 CSn | CS |
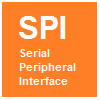
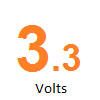
Photo
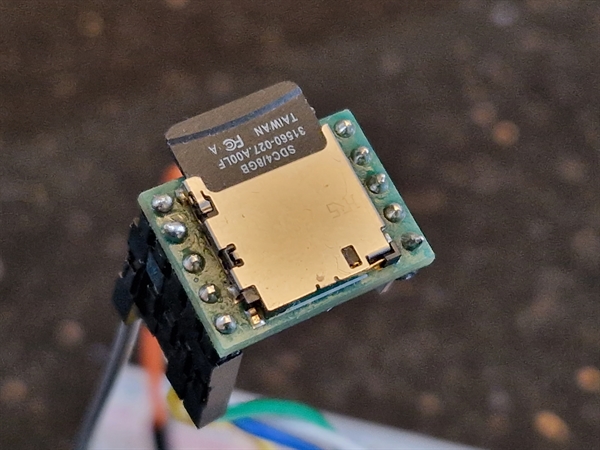
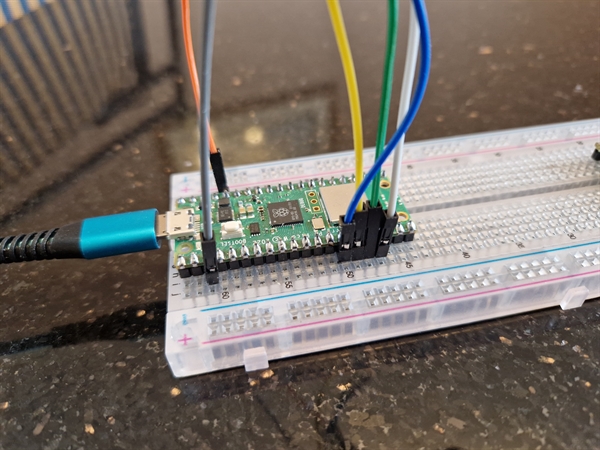
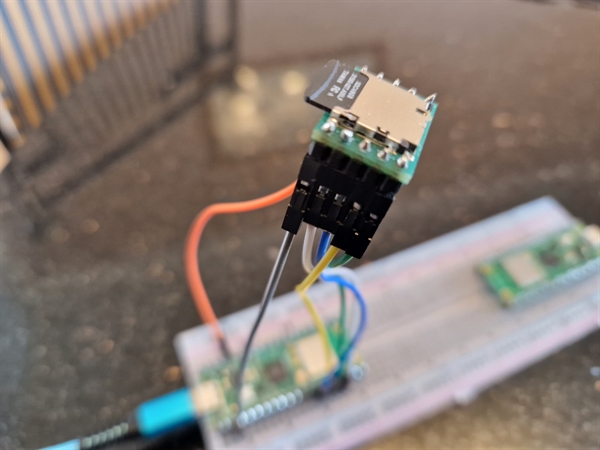
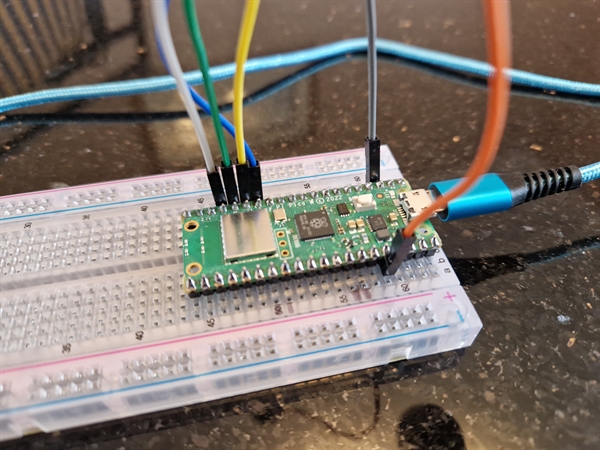
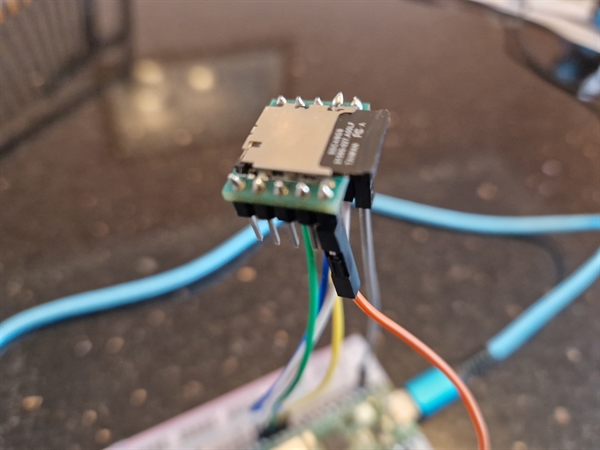
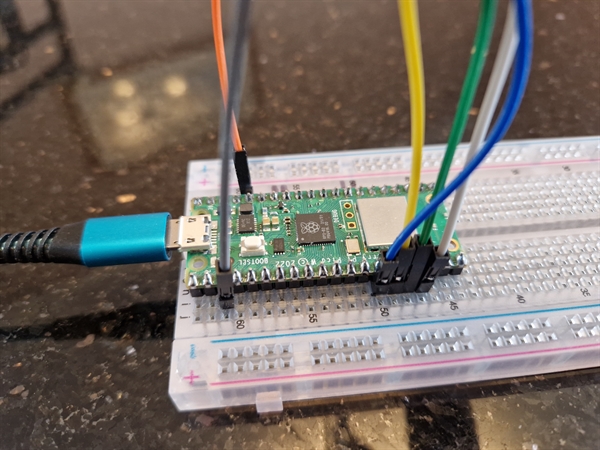