Raspberry Pi Pico W with GPS processed data
Example code of connecting the Raspberry Pi Pico W to a GPS module. Checking the checksum and extract some gps information.
Connections
Description | Colour | Pico W | GPS module |
---|---|---|---|
Power 3v | Orange | pin36 3V3(OUT) | 3.3v |
Ground | Black | pin3 GND | GND |
Pico W Transmit | Yellow | pin6 GP4 UART1_TX | GPS RX pin |
Pico W Receive | Green | pin7 GP5 UART1_RX | GPS TX pin |
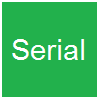
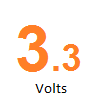
Photo
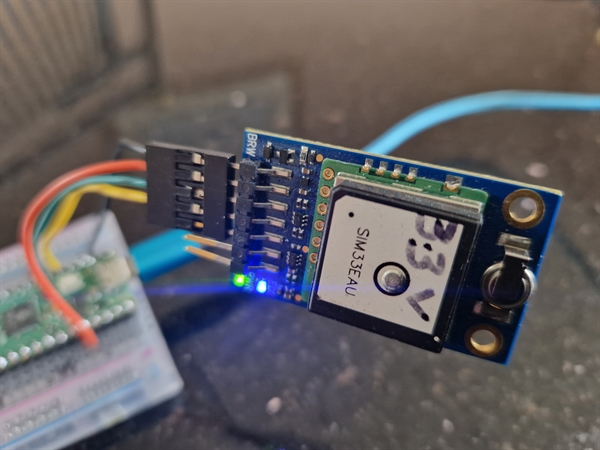
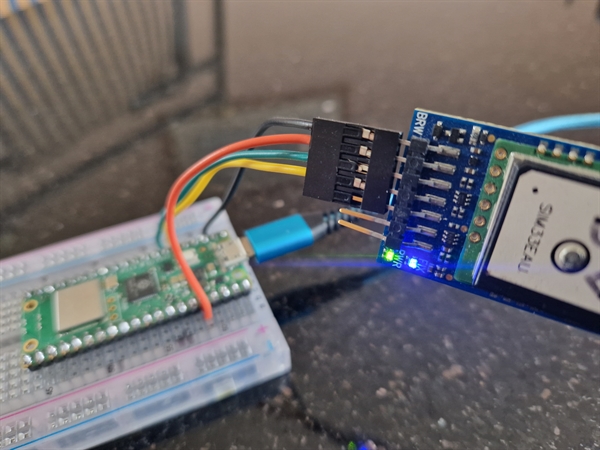
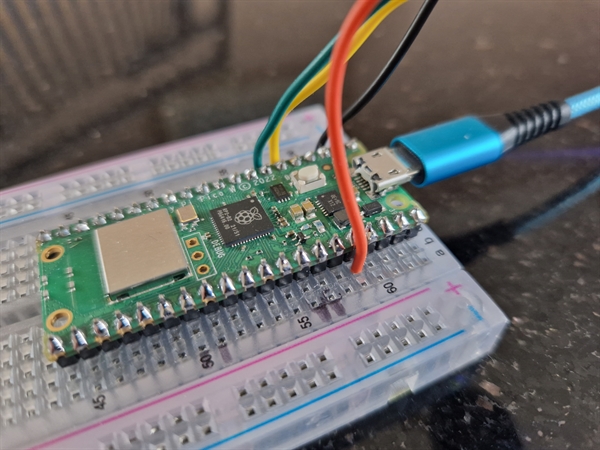
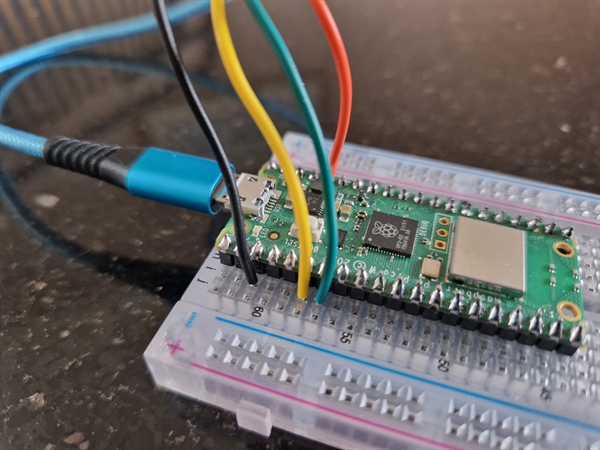