Raspberry Pi 3 + GPIO pins (File System)
Example code of connecting the Raspberry Pi v3 to a LED light. I'm using the Linux file system to control the GPIO pins, which is probably not ideal. The resistor is 220 ohms.
Photo
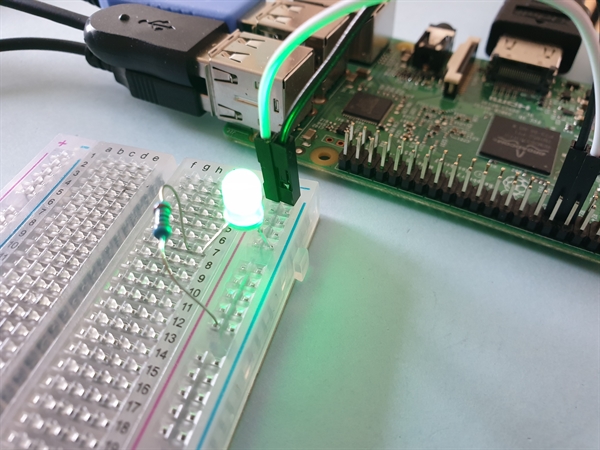
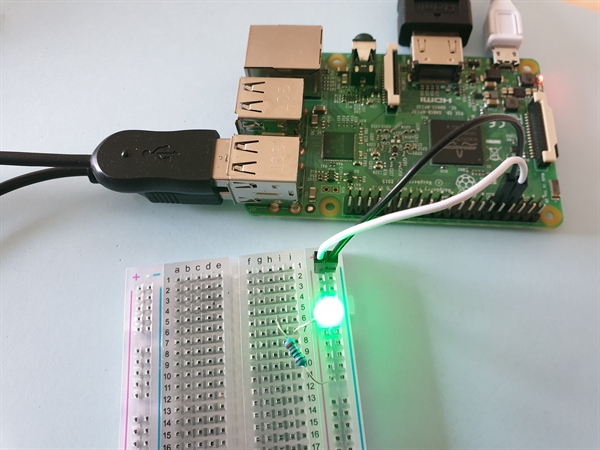
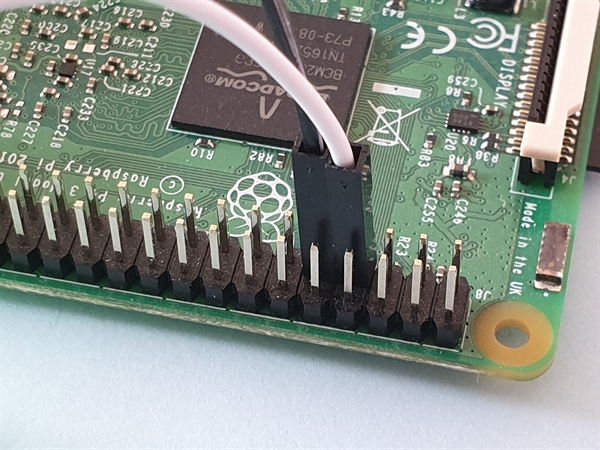
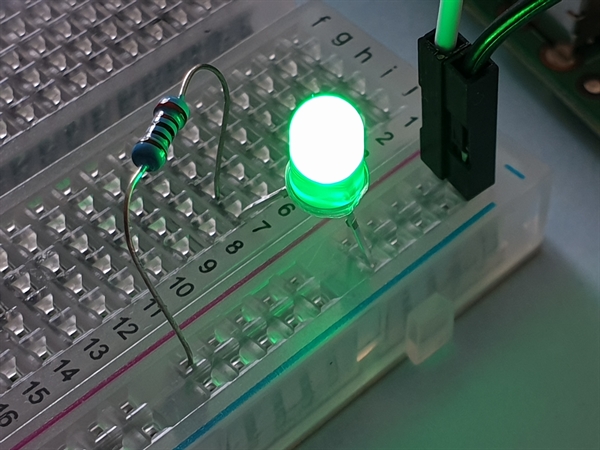
Example code of connecting the Raspberry Pi v3 to a LED light. I'm using the Linux file system to control the GPIO pins, which is probably not ideal. The resistor is 220 ohms.