BBC Microbit + Secure Digital
I feel it is always good to save my code variables, so I can go back and debug or understand better the robot behaviour. Now progress into wireless communication, I still see the benefit of saving observations locally on the robots. Partly because if communication is lost, I still have a black-box taking readings. I also don't have a computer by the side of the lake to store observations.
Photo
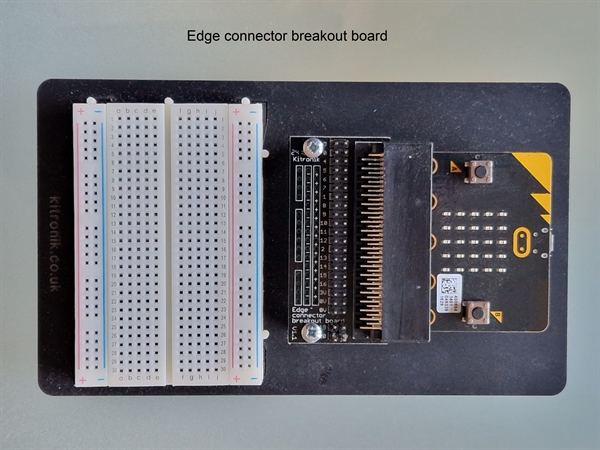
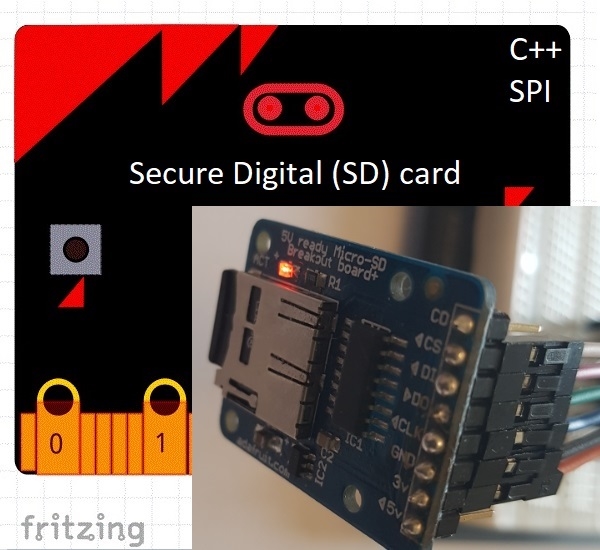
Sketch
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
// BBC Micro:bit writing to a Secure Digital (SD) device | |
// Copyright (C) 2019 https://www.roboticboat.uk | |
// e14977a4-9eaa-4740-a9e7-6dd329c255d8 | |
// | |
// This program is free software: you can redistribute it and/or modify | |
// it under the terms of the GNU General Public License as published by | |
// the Free Software Foundation, either version 3 of the License, or | |
// (at your option) any later version. | |
// | |
// This program is distributed in the hope that it will be useful, | |
// but WITHOUT ANY WARRANTY; without even the implied warranty of | |
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the | |
// GNU General Public License for more details. | |
// | |
// You should have received a copy of the GNU General Public License | |
// along with this program. If not, see <https://www.gnu.org/licenses/>. | |
// These Terms shall be governed and construed in accordance with the laws of | |
// England and Wales, without regard to its conflict of law provisions. | |
#include <SPI.h> | |
#include <SD.h> | |
#define chipSelectCS 16 | |
// CLK - pin 13 - Blue - Serial Clock managed by Micro:bit (Hardware pin) | |
// MISO - pin 14 - Green - Master input, Slave output (Hardware pin) | |
// MOSI - pin 15 - Purple - Master output, Slave input (Hardware pin) | |
// CS - pin 16 - White - Chip Select - Slave selected from available slaves | |
// VCC - v3.3 - Orange - Power supply | |
// GND - ground - Black - Ground | |
// Timer | |
unsigned long mytime; | |
void setup() | |
{ | |
// Update the User | |
Serial.begin(9600); | |
Serial.println("Initializing SD card..."); | |
// All SPI slaves are listening on the SPI | |
// Pin to tell our SPI device we want to communicate with it | |
pinMode(chipSelectCS, OUTPUT); | |
// Set pin to low, meaning we want to send data to this slave | |
digitalWrite(chipSelectCS, LOW); | |
// Is the SD device working ok? | |
if (!SD.begin(chipSelectCS)) | |
{ | |
// SD device has a problem | |
Serial.println("SD failed"); | |
delay(1000); | |
return; | |
} | |
} | |
void loop() | |
{ | |
// Update the time | |
mytime = millis(); | |
// Open the file to write to | |
File dataFile = SD.open("datalog.txt", FILE_WRITE); | |
// Was the file opened ok? | |
if (dataFile) | |
{ | |
// Write the time to the disk | |
dataFile.println(mytime); | |
//Close the file | |
dataFile.close(); | |
// Update User of progress | |
Serial.println(mytime, DEC); | |
} | |
else | |
{ | |
// File could not be opened. Error :-( | |
// On PC, clear the contents of the datalog.txt and try again | |
Serial.println("error opening datalog.txt"); | |
delay(50); | |
} | |
} | |